Asynchronous JavaScript
Async is another hard pard to understand how JavsScript works. In this article, we discover why we need asynchronous, how asynchronous works, use asynchronous functions -- promise, stop a function run with Generator and improve asynchronous code with async-await.
Why we need asynchronous
JavaScript is single-threaded, goes through the code line-by-line, and runs/ executes each line; it never moves to the following line until finished on the previous line. So what if we need to wait some time before we can execute certain code? Like, fetch data from an API/server. We have to delay some code execution but not block the thread from any following code running while we wait. Asynchronous means later; asynchronous code doesn't run now but as sometime later.
How asynchronous works
As I mentioned in a previous post JavaScript engine has three parts: Thread of execution, Memory/variable environment, Call stack. This engine can't run asynchronous code. We need another part: a queue. This queue is used for waiting for stack empty. One stack is empty; push a function to stack. Imaging we fetch data from a server, after 1-second data return from server, browsers add a function to queue, then push to stack and run it one stack is empty. This prosecco is called Event Loop.
note: event loop is part of HTML Specifications, not JavaScript
Zero delays
delay zero seconds don't not run Immediately but add to queue immediately, then wait for call stack empty
function print(){ console.log("Hello"); }
function wait1Second(){ //block thread for1 second }
setTimeout(wait1Second,0);
blockFor1Sec()
console.log("Me first!")
before execution, the compiler creates global scope and function's scope; at execution time, JavaScript engine initiates global scope by adding declarations to memory, variable define initializes to 'undefined,' function declaration assigning function along with its scope
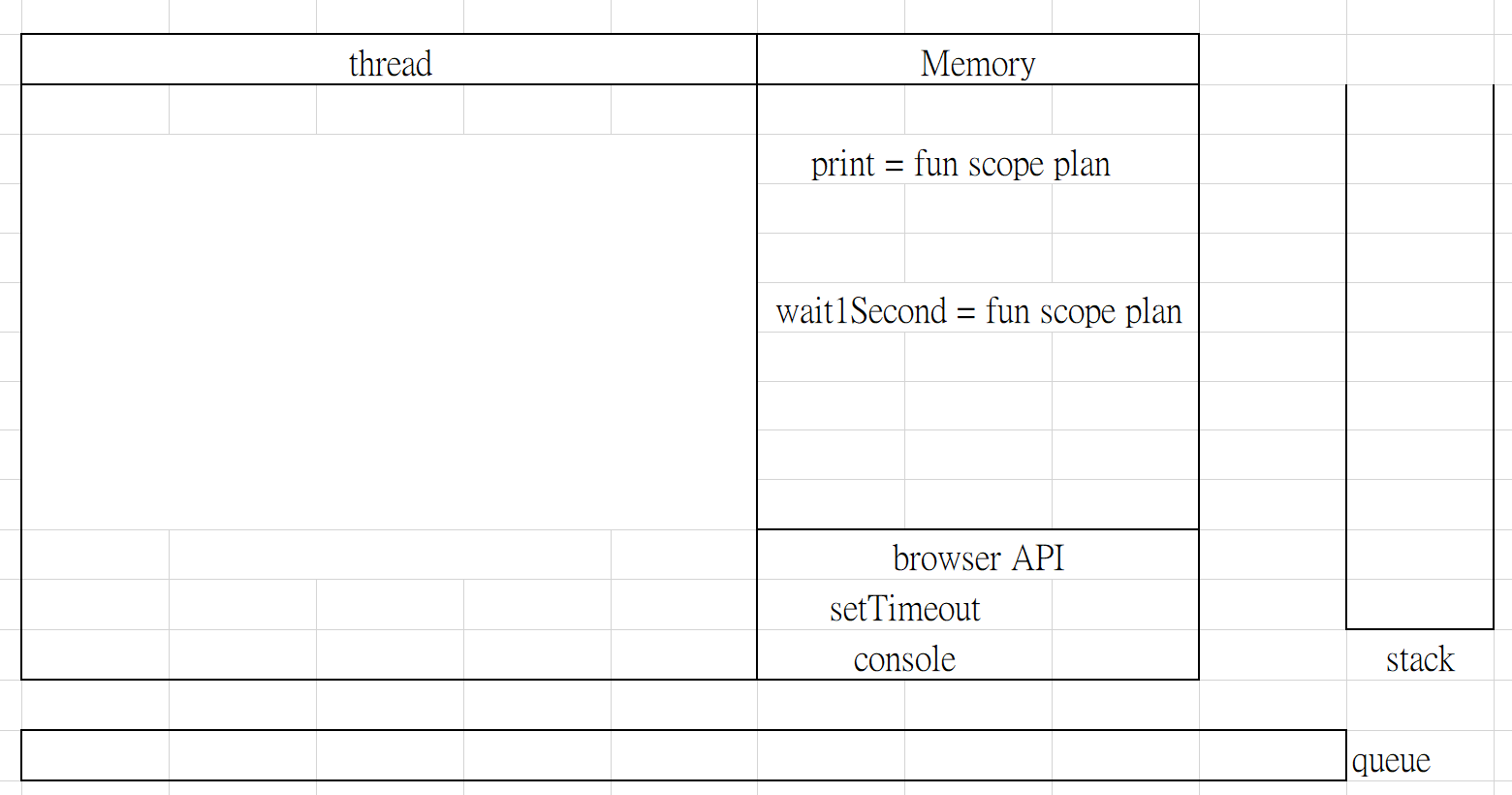
setTimeout
is a browser API that executes a function after a timer expires. When invoking, browser set up a timer in the background. Then adds a function to the queue after time elapsed.
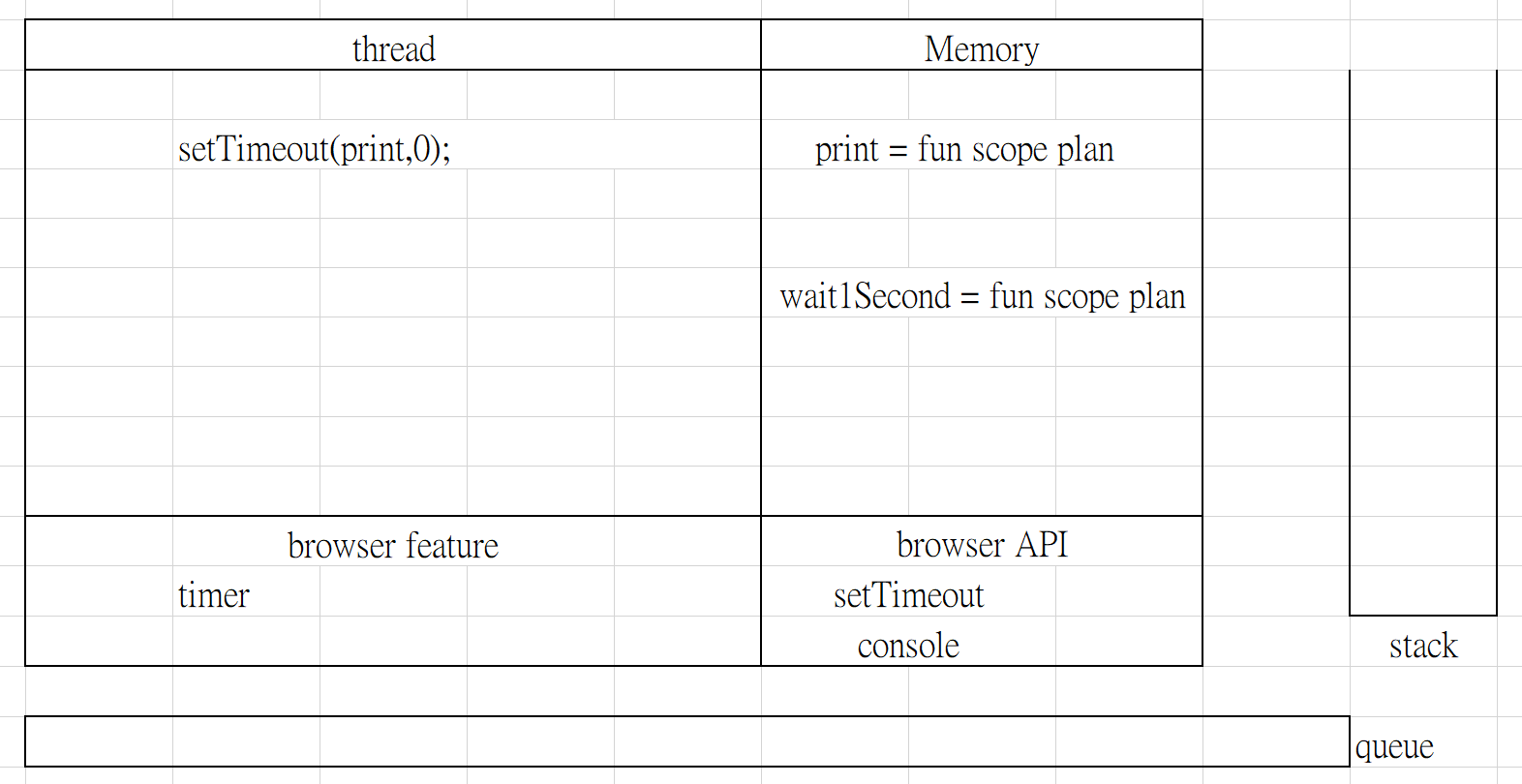
wait1Second
simulate a function that takes one second to finish. Because one second is greater than zero seconds, time expires will JavaSript run this function, the print function added to the queue.
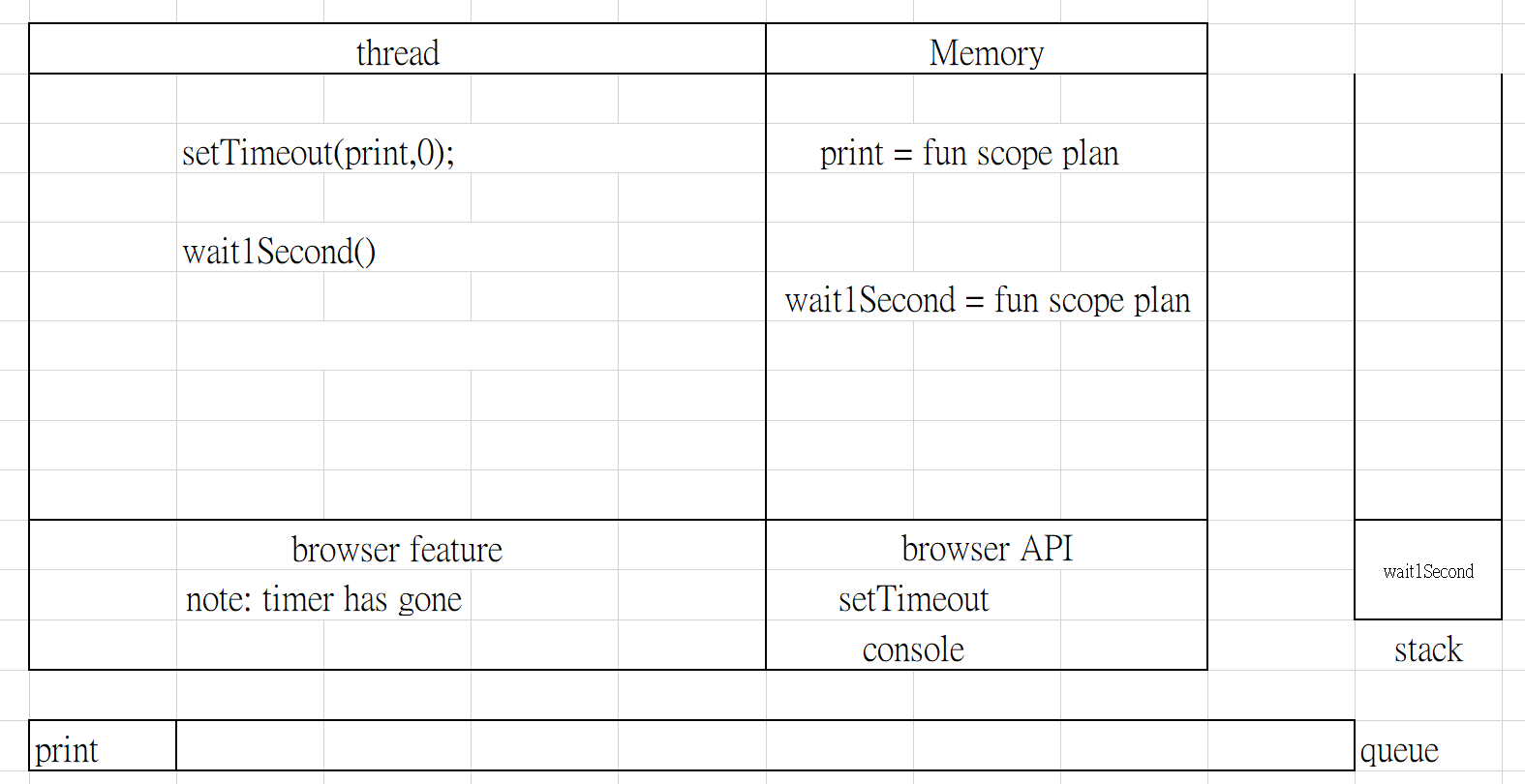
console log is synchronous, so it shows before async
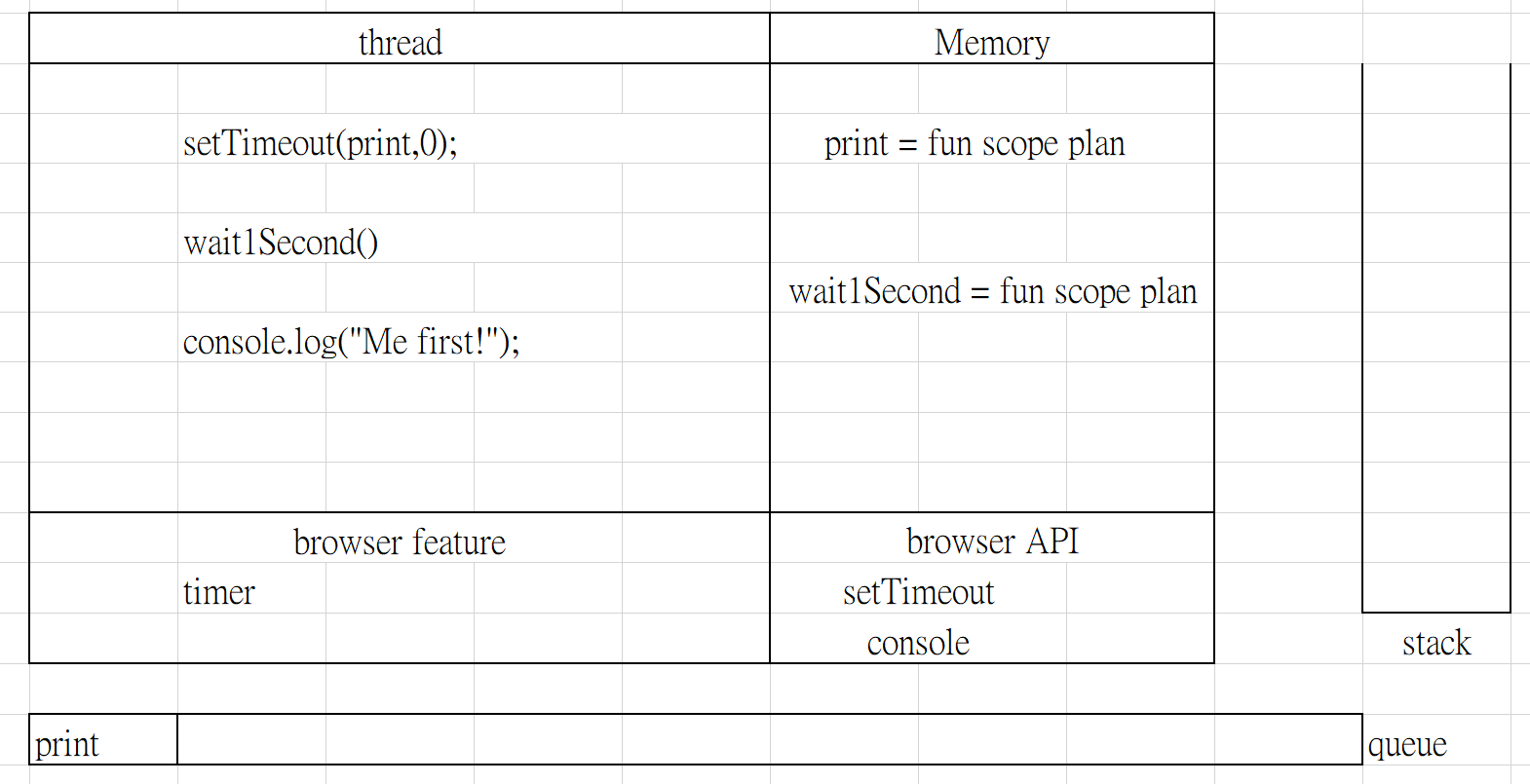
All synchronous had ran. The stack is empty. even loop add print to call stack, then create an execution context for print function
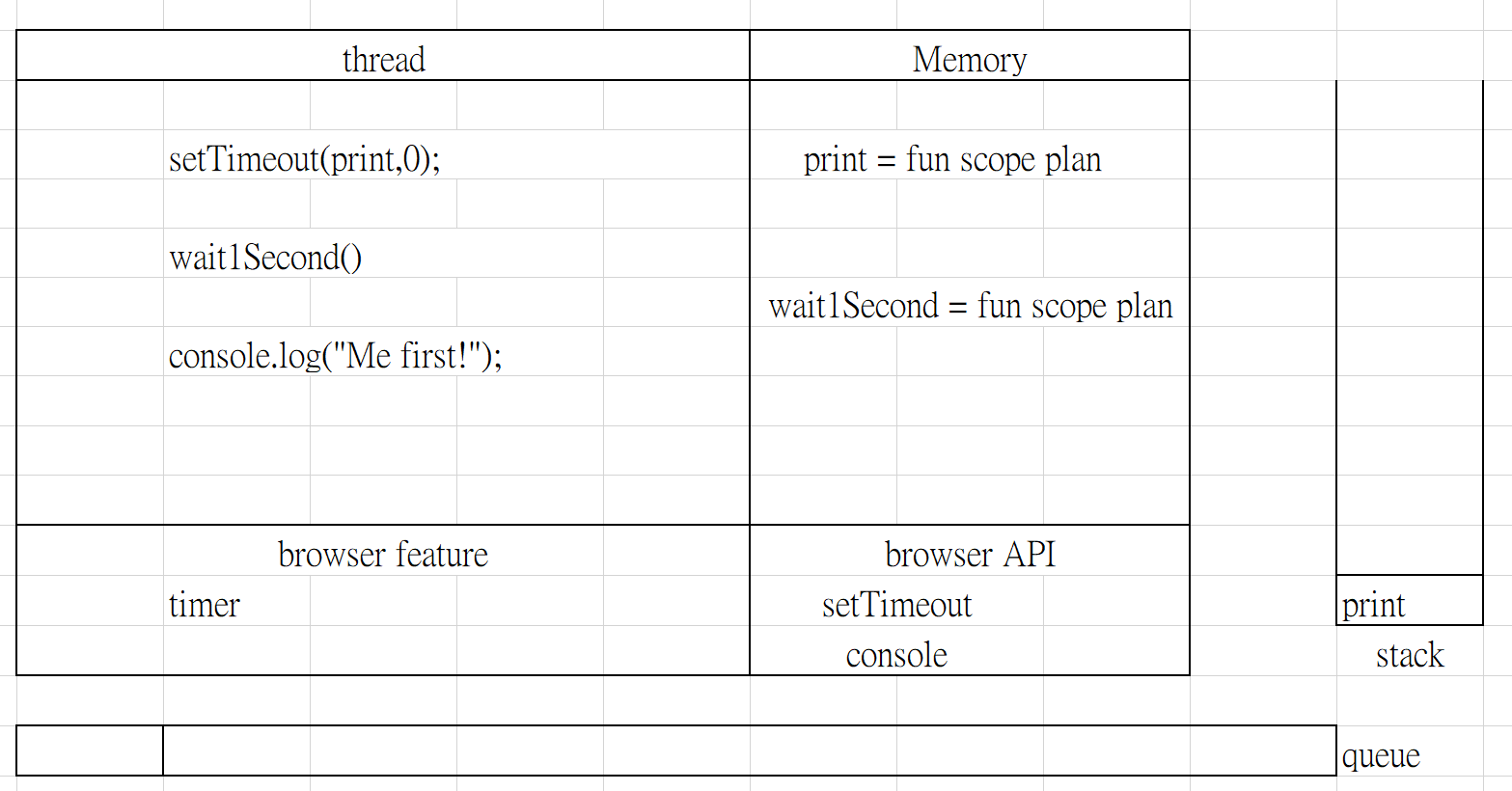
Promise
promise is another async API that represents a future along with a then function defers code running until some background work is done. The difference async behavior from other API is function pass to then method will add to a specific queue called Job queue
. Other functions are added to the callback queue
. Job queue has high priority than call queue means the function in callback queue executes after job queue is empty.
function print(data){console.log(data)}
function printSecond(){console.log(“second”);}
function wait1Second(){ //block thread for1 second }
setTimeout(printSecond, 0);
new Promise((res, rej) => res('first')).then(print)
wait1second()
the execution processs is look like this. (I spik serveral step here). all asyn function are waiting in queue, but function in Job queue add to call stack first.
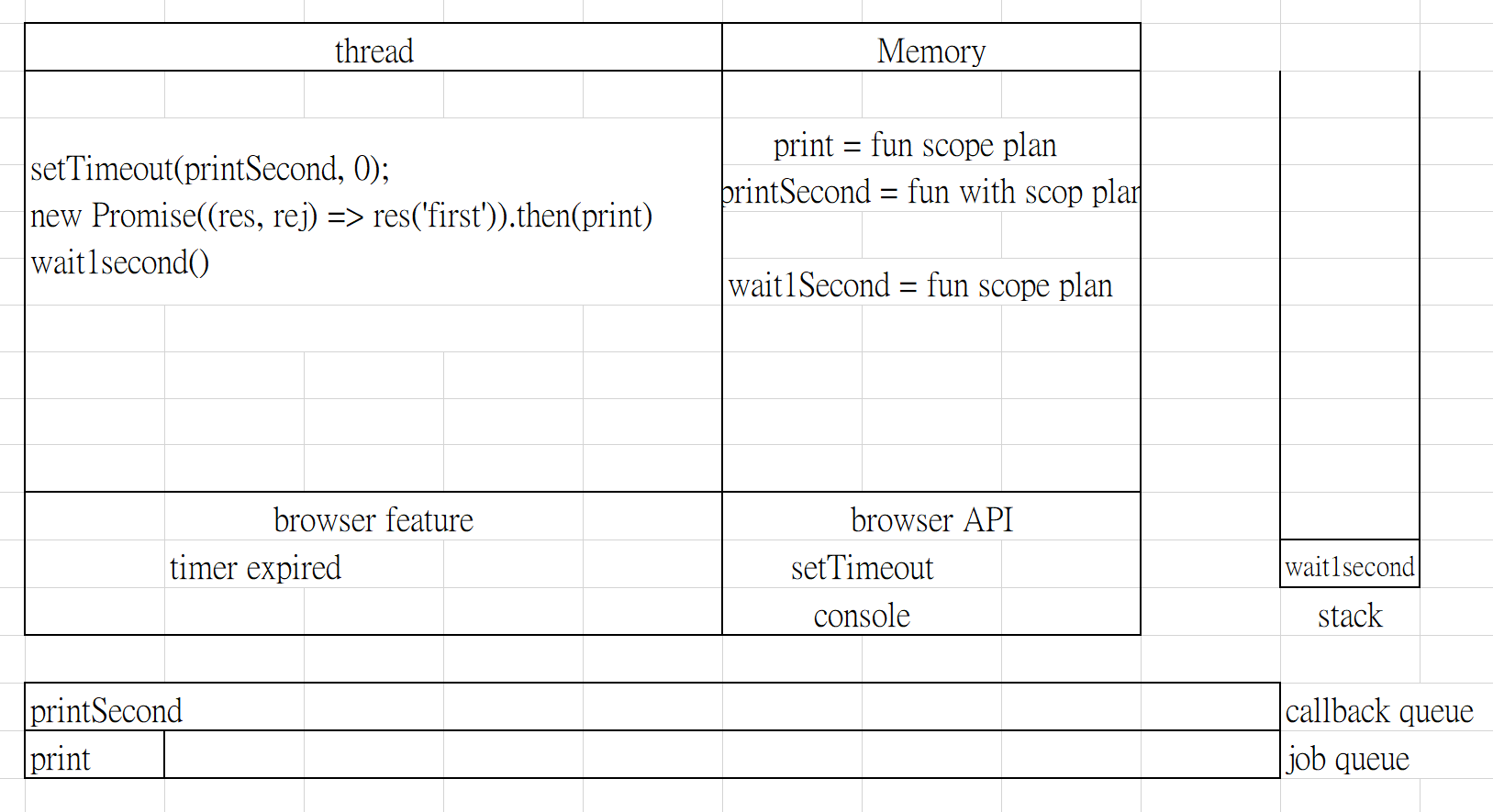
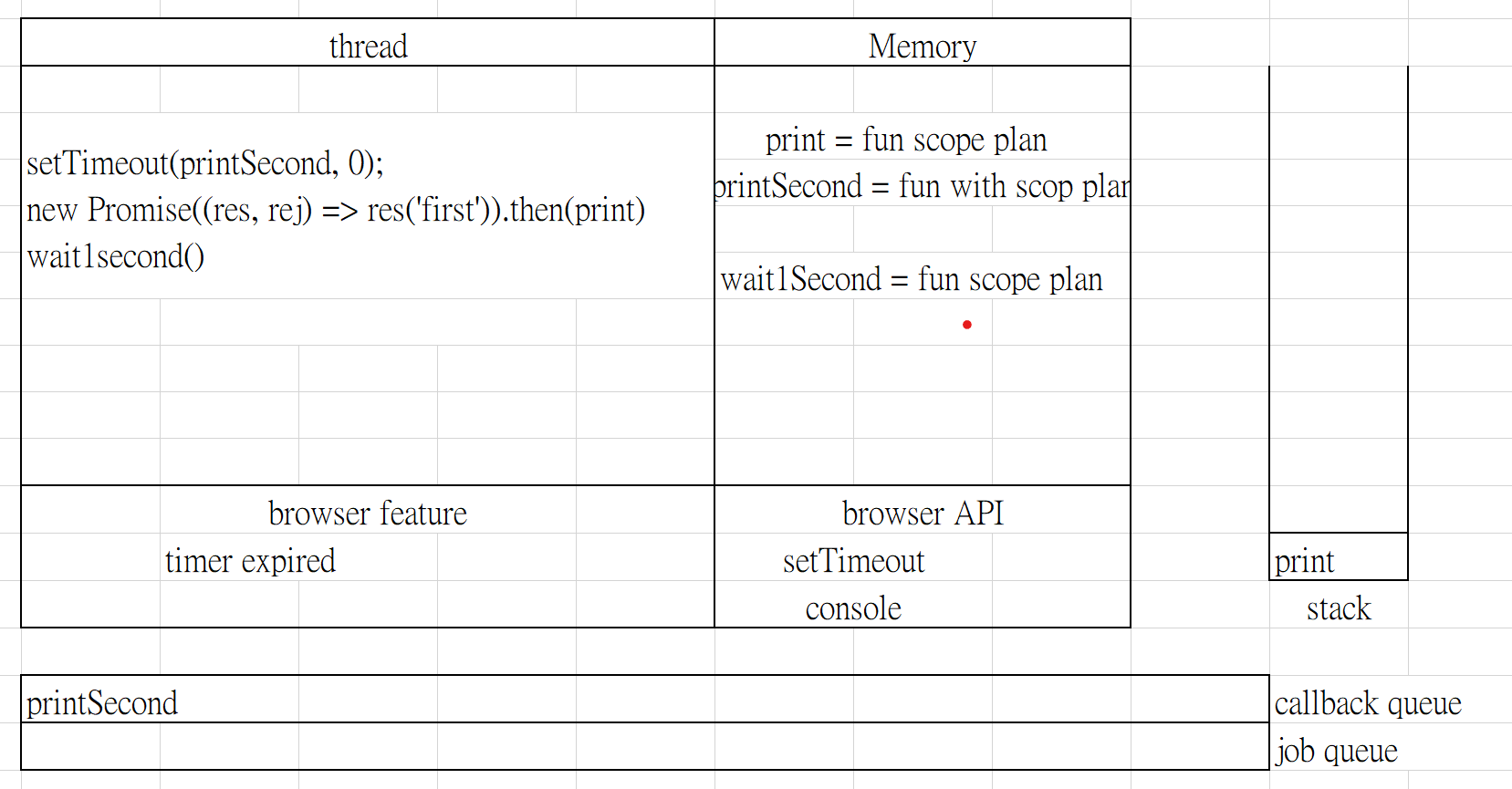
Summary asynchronous execution. Promise async function hold in job queue and non-promise async function hold in callback queue; when the call stack is totally empty adding a function to call stack; Job Queue has high priority than callback stack
Generator
to do